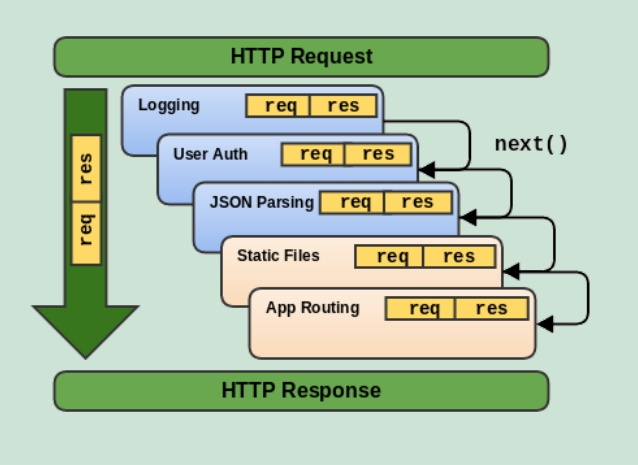
In modern web development, handling HTTP requests efficiently and securely is crucial. Express.js, a popular framework for Node.js, offers a robust solution for this through its body parser middleware. Today we’ll drive deeper into the body parser middleware and its significance in backend development.
What is Body Parser Middleware?
Body parser middleware is an essential component in Express applications. It processes incoming request bodies, making it easier to handle POST
and PUT
requests. By parsing the body of an HTTP request and attaching it to the req.body
property, it simplifies data extraction and manipulation in server-side logic.
How Does it Work?
When a client sends a request to the server, the body parser middleware intercepts this request. It parses different types of payloads, such as URL-encoded data or JSON, and then populates the req.body
object with parsed data. This process turns the raw HTTP request body into a format that’s more convenient to work with in JavaScript.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// parse application/json
app.use(bodyParser.json());
app.post('/submit-data', (req, res) => {
console.log(req.body); // Access the parsed body here
res.send('Data Received');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
3. Practical Applications
The body parser middleware is particularly useful in scenarios where you need to process form data or handle JSON payloads in API requests. For instance, when building RESTful APIs, it ensures that the data sent by the client is correctly parsed and ready for processing by backend logic.
Conclusion
The body parser middleware in Express streamlines the handling of HTTP request bodies, making backend development more efficient and secure. Understanding its role and implementation is key for developers looking to build robust and scalable web applications with Node.js and Express.
,