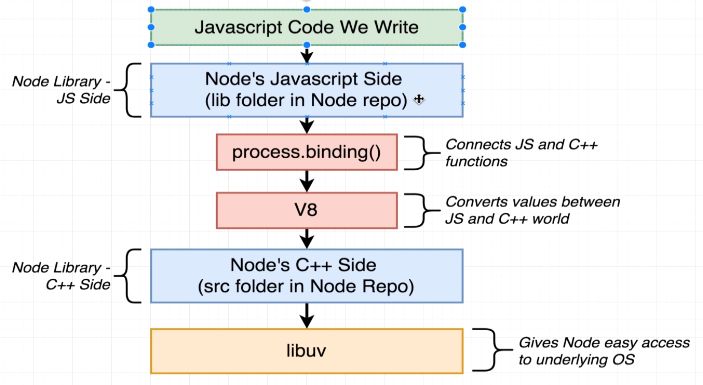
Node.js has become one of the most popular environments for building web applications, APIs, and more. However, to fully utilize its power, it’s crucial to understand what happens under the hood. In this article, we’ll explore how Node.js works, touching on aspects like its architecture, V8 engine, libuv, and more.
Architecture Overview
The Node.js environment comprises several layers:
- Javascript Code We Write: The code you write for your applications.
- Node’s JavaScript Side: Core libraries written in JavaScript.
- Node’s C++ Side: Core functionalities implemented in C++.
- V8 Engine: The JavaScript execution engine.
- Libuv: A multi-platform support library for asynchronous I/O.
The JavaScript Side and process.binding
Node.js extends JavaScript capabilities by providing built-in modules (like fs
for file handling, http
for HTTP requests, etc.). These modules are present in the ‘lib’ folder of the Node.js source code and are written in JavaScript. However, they often need to communicate with the underlying system, which is where process.binding
comes in.
The process.binding
method allows these modules to bridge into C++ code, essentially allowing JavaScript to execute lower-level operations that it would not usually be able to perform.
V8 Engine
V8 is Google’s open-source JavaScript engine that converts JavaScript code into lower-level machine code that your computer can execute. Node.js uses V8 to execute the JavaScript code you write.
Node’s C++ Side
This part of Node is where the real magic happens. Here, native bindings written in C++ interact with the system at a low level, performing operations like file I/O, network communications, etc. The C++ layer is what allows Node.js to be incredibly efficient and performant.
Libuv
Libuv is the library that gives Node.js its event-driven, non-blocking I/O model. Written primarily for Node.js, libuv provides an abstraction around libev (event library) or directly around the native event mechanisms, depending on the platform.
Connecting the Dots: Node Library
The Node library acts as a bridge between the JavaScript and C++ worlds in several ways:
- Connects JS and C++ functions: When you call a Node.js API from your code, it eventually maps to a C++ function that executes the underlying operation.
- Converts values between JS and C++: Data types used in JavaScript and C++ may not always align. The Node library handles the translation between these worlds.
- Provides Access to the OS: Many Node.js functions require direct access to the operating system, like reading from the file system or opening a network socket. Node.js, through its native bindings, allows this to happen seamlessly.
Reading a File in Node.js
Let’s consider reading a file in Node.js to see the interaction between these layers.
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Here’s what happens under the hood:
- Your JavaScript code calls
fs.readFile
. - The
fs
module, part of Node’s JavaScript library, usesprocess.binding
to call the appropriate C++ function. - The C++ function, powered by libuv, performs the file reading operation asynchronously.
- Once the operation is complete, the callback function you provided is executed, presenting you with the file data.
Conclusion
Understanding Node.js at this level enables you to appreciate the intricacies involved in executing even the simplest operations. Whether you’re a beginner just getting started or a seasoned developer aiming to optimize your applications, this deep dive into how Node.js works under the hood should provide you valuable insights.
,