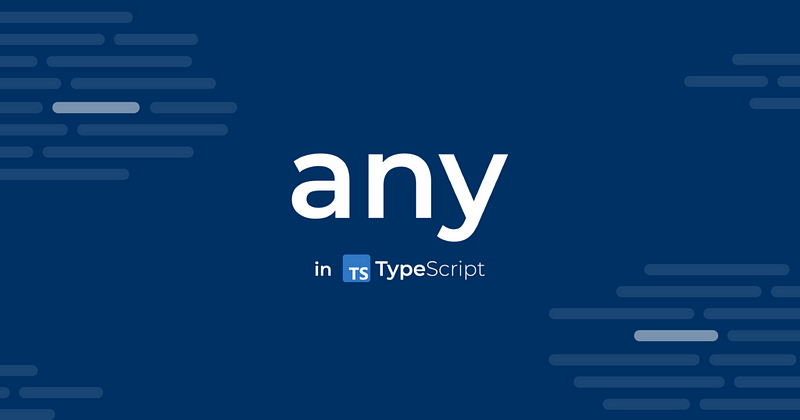
In TypeScript, types are crucial for catching errors during development, enhancing code readability, and ensuring code quality. However, there exists a special type called any
, which, as the name suggests, allows a variable to be of any type. While it might seem like a flexible option, it essentially turns off TypeScript’s type checking for that variable. In this article, we’ll explore what the any
type is, why you should be cautious while using it, and what are the alternatives.
What is the any
Type?
The any
type is a type in TypeScript that signifies that a variable can hold values of any type—string
, number
, boolean
, object
, and so on. When a variable is of type any
, TypeScript refrains from type-checking operations on that variable.
let anything: any = 42;
anything = 'hello';
anything = [1, 2, 3];
Why Should You Avoid any
?
Loss of Type Safety
TypeScript’s core advantage is its type system. When you use any
, you are essentially opting out of the benefits TypeScript provides, such as type-checking, autocompletion, and more.
Error Prone
With the any
type, TypeScript can’t check for correct property references, which makes the code more prone to runtime errors.
let something: any = 'hello world';
something.foo(); // TypeScript won't catch this mistake
Poor Readability
The use of any
can reduce code readability by not providing enough information about the variable’s intended use, making it harder for other developers to understand the code.
Code Maintenance
Overuse of any
can lead to code that is harder to refactor, debug, or extend, especially in large codebases.
Alternatives to any
Use Type Annotations
Whenever possible, use explicit type annotations to indicate what type a variable should be.
let username: string = 'JohnDoe';
Use Union Types
If a variable can be one of multiple types, use a union type.
let id: string | number = '123';
id = 123;
Use Generics
For functions and classes that work with different types, consider using generics.
function echo<T>(value: T): T {
return value;
}
Conclusion
While the any
type in TypeScript provides flexibility, it comes at the cost of losing type safety, making the code error-prone, and reducing maintainability. Therefore, it’s advisable to avoid using any
wherever possible and to take advantage of TypeScript’s robust type system for a more secure and manageable codebase.
,