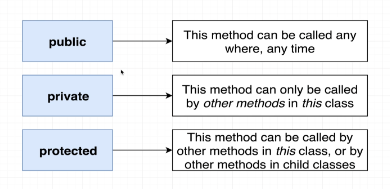
TypeScript enhances JavaScript’s object-oriented programming capabilities by adding features like classes and access modifiers. Class access modifiers in TypeScript help control the visibility and accessibility of class members (properties, methods) within a program. The three primary access modifiers are public
, private
, and protected
.
Public Modifier
The public
modifier is the most permissive access level. When a class member is marked as public
, it can be accessed from anywhere, whether inside the class, from instances of the class, or even from derived classes. If you don’t set a modifier, it is automatically assigned public.
class Animal {
public name: string;
constructor(name: string) {
this.name = name;
}
public makeSound() {
console.log("Generic animal sound");
}
}
const dog = new Animal("Dog");
console.log(dog.name); // Output: "Dog"
dog.makeSound(); // Output: "Generic animal sound"
Private Modifier
The private
modifier restricts access to class members. A private
member can only be accessed by other methods within the same class.
class Human {
private socialSecurityNumber: string;
constructor(ssn: string) {
this.socialSecurityNumber = ssn;
}
getSSN() {
return this.socialSecurityNumber;
}
}
const john = new Human("123-45-6789");
console.log(john.getSSN()); // Output: "123-45-6789"
console.log(john.socialSecurityNumber); // Error: Property 'socialSecurityNumber' is private and only accessible within class 'Human'.
Protected Modifier
The protected
modifier is like private
, but it extends the accessibility to derived (or child) classes. Members marked as protected
can be accessed by methods in the same class and methods in derived classes.
class Vehicle {
protected speed: number;
constructor(speed: number) {
this.speed = speed;
}
}
class Car extends Vehicle {
constructor(speed: number) {
super(speed);
}
public showSpeed() {
console.log(`The speed is ${this.speed} mph.`);
}
}
const myCar = new Car(120);
myCar.showSpeed(); // Output: "The speed is 120 mph."
Conclusion
Understanding the different access modifiers — public
, private
, and protected
—is crucial for effective TypeScript programming. They provide a way to encapsulate data and control what can be accessed and modified, making it easier to manage large codebases and collaborate with other developers.
,