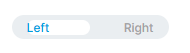
If you need to create a toggle button component in react, the quickest possible way to do it is by using the react-switch library. Check out my other tutorial which uses the react-switch library.
If you need a button with a bit more styling, this tutorial is for you. We’ll use React Styled Components library which allows us to write actual CSS within our Javascript code.
First, create the ToggleButton component, and import the stylesheet from a separate file called styles:
import React, { useState } from 'react';
import * as S from './styles';
const ToggleButton = ({ value, setValue }) => {
const [value, setValue] = useState(false);
const handleClick = () => {
setValue(!stateValue);
setStateValue(!stateValue);
};
return (
<S.Wrapper>
<S.Button active={value} onClick={handleClick}>
<S.LeftText active={value}>Left</S.LeftText>
<S.RightText active={value}>Right</S.RightText>
</S.Button>
</S.Wrapper>
);
};
export default ToggleButton;
Then, install the styled-components library.
npm install --save styled-components
Out styles file will look like this, make sure you import the styled-components library.
import styled from 'styled-components';
export const Wrapper = styled.div`
display: flex;
align-items: center;
justify-content: space-between;
position: relative;
`;
export const Button = styled.button`
display: flex;
align-items: center;
justify-content: space-between;
width: 157px;
height: 24px;
color: white;
border: none;
border-radius: 15px;
padding: 0 15px;
background-color: #eaeef1;
`;
export const LeftText = styled.div`
flex: 1;
width: 30%;
border-radius: 15px;
text-align: left;
font-family: Inter;
font-style: normal;
font-weight: 500;
font-size: 12px;
line-height: 15px;
z-index: 1000;
color: ${props => (props.active ? '#9FA1A3' : '#009CDF')};
background-color: ${props => (props.active ? '#eaeef1' : 'white')};
`;
export const RightText = styled.div`
flex: 1;
width: 30%;
text-align: right;
color: green;
border-radius: 15px;
font-family: Inter;
font-style: normal;
font-weight: 500;
font-size: 12px;
line-height: 15px;
z-index: 1000;
color: ${props => (props.active ? '#009CDF' : '#9FA1A3')};
background-color: ${props => (props.active ? 'white' : '#eaeef1')};
`;
We pass our state variable as a prop into our styled components, this allows us to render some styles conditionally such as the colour of the text and background.