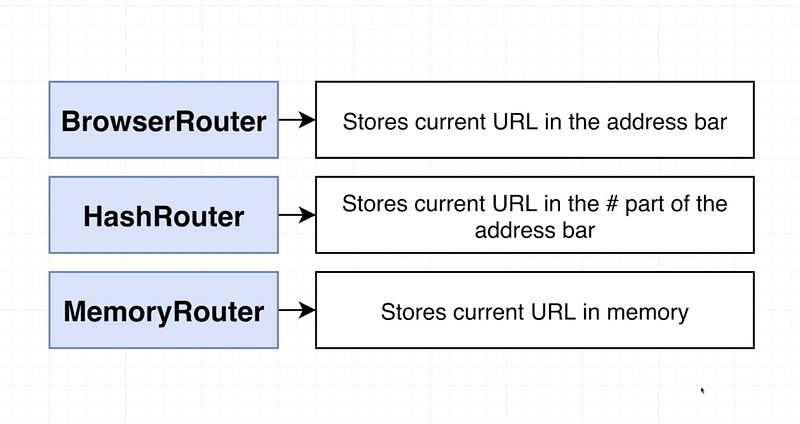
Introduction
React Router plays a crucial role in building single-page applications (SPAs) with React. It helps manage the navigation and UI state of the application. In React Router, there are mainly three types of routers: BrowserRouter
, HashRouter
, and MemoryRouter
. This article provides a concise overview of each.
1. BrowserRouter
Purpose
BrowserRouter
is the most commonly used router in React applications. It utilizes the HTML5 history API to keep your UI in sync with the URL.
When to Use
- For modern web applications where clean URLs are preferred.
- When your server supports all URLs pointing to
index.html
, allowing React to manage the routing.
Example
import { BrowserRouter, Route } from 'react-router-dom';
const App = () => (
<BrowserRouter>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</BrowserRouter>
);
2. HashRouter
Purpose
HashRouter
uses the URL’s hash portion to keep your UI in sync with the URL.
When to Use
- For older browsers or environments where the server doesn’t support client-side routing.
- When you need to serve static files and don’t have control over server configuration.
Example
import { HashRouter, Route } from 'react-router-dom';
const App = () => (
<HashRouter>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</HashRouter>
);
3. MemoryRouter
Purpose
MemoryRouter
doesn’t use the URL for routing. Instead, it keeps the routing history internally in memory.
When to Use
- For testing and non-browser environments like React Native.
- When you want to control navigation programmatically.
Example
import { MemoryRouter, Route } from 'react-router-dom';
const App = () => (
<MemoryRouter initialEntries={['/','/about']} initialIndex={0}>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</MemoryRouter>
);
Conclusion
Understanding the key differences between BrowserRouter
, HashRouter
, and MemoryRouter
allows you to make informed decisions about routing in your React applications. Choose the one that best fits your application’s needs and constraints for a smooth user experience.
,